Working with the Reflect API in JavaScript
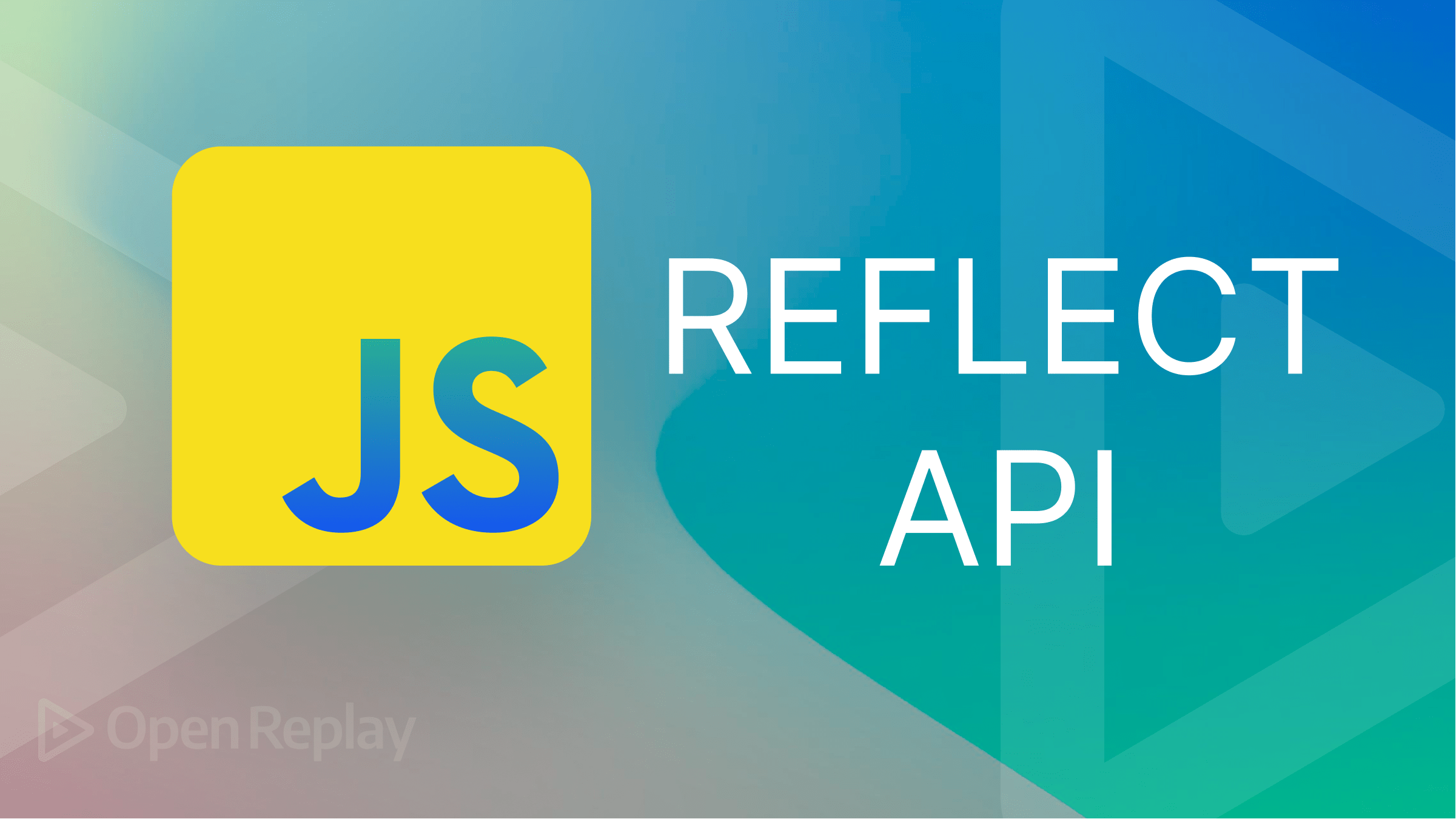
If you are reading this article, the question in your mind is probably, “What does this Reflect
API do?”
In this article, we will understand the concept of Reflect
in JavaScript, and discover some of the methods available in the Reflect
API and how they can be used to improve your code in real-world scenarios.
Whether you are a beginner or an experienced developer, this article will provide a clear guide to working with the Reflect
API and help you take your JavaScript skills to the next level.
Definition of JavaScript Reflect
API
The Reflect
API in JavaScript is an inbuilt ES6 global object that allows you to perform various tasks related to interacting with and manipulating JavaScript objects, such as setting and getting property values, creating and defining new objects, and more.
Think of the JavaScript Reflect
API as a toolbox with different tools (methods) that helps you understand and control JavaScript objects. The Reflect
API helps us open the box (object), look inside, and even change what is inside. It also helps us figure out information about the box (object), like how many things are inside and what kind of box it is.
The Reflect
API is particularly useful in modern JavaScript development. It makes your code easier to read and understand, which is important when working on large or complex projects. It can also help you avoid mistakes.
How to use the reflect
API
The Reflect
API is built into JavaScript, so you don’t need to import
or install
any library in your code for the Reflect
API to work if it’s already supported in your JavaScript environment. The Reflect
API is part of the JavaScript language itself and is available in modern JavaScript environments, such as modern browsers and recent versions of Node.js. To use the Reflect
API, simply call the desired method, such as Reflect.get()
, Reflect.set()
, etc.
Here is an example of how you might use the Reflect
API in your code if it’s already supported:
// file: index.js
let obj = {name: 'Solomon', class: 2}
let value = Reflect.get(obj, 'name')
console.log(value)
// in terminal
$ node index.js
Solomon //this is the result of the above code
The image below this text provides a pictorial view of what the information discussed looks like on my computer.
Here is the result at the terminal
In this example, the Reflect
API is used directly, without any additional setup or imports. If your version of Node.js supports the Reflect
API, this code should work without any issues. Therefore, you must have Node.js
installed on your computer.
Session Replay for Developers
Uncover frustrations, understand bugs and fix slowdowns like never before with OpenReplay — an open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data. Check our GitHub repo and join the thousands of developers in our community.
Commonly used Reflect
API
These are the most commonly used methods in the API.
Reflect.get()
The Reflect.get()
method allows you to get the value of an object’s property. Think of it like a tool that helps you get a specific item from a box. Here’s how it works with an example:
// file: index.js
let person = { name: 'Solomon', class: 4};
let name = Reflect.get(person, 'name')
console.log(name); // Result: Solomon
// in terminal
$ node index.js
Solomon //Result
In this example, we have an object person
with two properties, name
, and class
. We use Reflect.get
method to get the value of the name
. Then, we log the value of the name
to the terminal, which is the output called Solomon
.
NOTE: The approach of using the Reflect.get(person, 'name')
is that this method will return a value undefined
if the property is not found, which is useful for debugging.
Here is an example:
// file: index.js
let person = { name: 'Solomon', class: 4};
let address = Reflect.get(person, 'address')
console.log(address); // Result: undefined
// in terminal
$ node index.js
undefined //Result
The Reflect.get()
method works by taking in two arguments:
target
: The object to target.propertykey
: The name of the property you want to get.
This method has an optional third argument, the;
receiver
(optional): This argument allows you to specify the object that should be treated as thethis
object when the property is accessed.
Reflect.set()
The Reflect.set()
method allows you to change the value of a property in an object.
Here is an example of how you can use Reflect.set()
:
// file: index.js
let person = { name: 'Solomon'};
Reflect.set(person, 'name', 'Jude');
console.log(person.name) //Result: 'Jude'
// in terminal
$ node index.js
Jude // Result
In the example above, we first created an object called person
with a property called name
and the value Solomon
. Then we used the Reflect.set() method to change the value of the name
property to Jude
. Finally, we logged the value of the name
property and saw that it was successfully changed to Jude
.
This method works by taking in three arguments:
target
: The object you want to set a property on.propertykey
: The name of the property you want to set.value
: The new value you want to set the property to.
This method also has an optional fourth argument, the;
receiver
(optional): This is the object that should be treated as thethis
object when the property is set.
Reflect.has()
The Reflect.has()
method is a way to check if an object has a certain property. It is like asking if an object has a specific thing. This method returns a Boolean
(true or false) value.
Here is an example to help illustrate this concept:
// file: index.js
let person = { name: 'Jude'};
let hasName = Reflect.has(person, 'name');
console.log(hasName);
// in terminal
$ node index.js
true //Result
In this example, the second line creates an object called person
with a property name
. The third line uses the Reflect.has()
method to check if the person
object has a property called name
. The result of this check is stored in a variable called hasName
.
Finally, the fourth line logs the value of hasName
, which is true
, because the person
object does have a property called name
, and if the object does not have the property, it returns false
Here is an example:
// file: index.js
let person = { age: 4};
let hasName = Reflect.has(person, 'name');
console.log(hasName);
// in terminal
$ node index.js
false //Result
This method takes two arguments:
target
: The object that you want to check for a property.propertykey
: The name of the property that you want to check.
Reflect.defineProperty()
The Reflect.defineProperty()
method is a way to add or change a property on an object. It is like adding a new toy to a box of toys or changing the color of a toy in the box. This method works by taking in three arguments:
target
: The object on which the property is to be defined or modified.propertykey
: The property’s name to define or modify.attributes
: The attribute of the property being defined.
Here is an example to help illustrate this concept:
//This is an example of how to use Reflect.defineProperty() method to define a new property on an object
let obj = {};
Reflect.defineProperty(obj, "name",{ value: "OpenReplay"});
console.log(obj.name);
//Result: "OpenReplay"
//This is an example of how to use Reflect.defineProperty() method to modify an existing property on an object
let obj = { name: "Solomon Jude"};
Reflect.defineProperty(obj, "name", { value: "Solomon Franklin"});
console.log(obj.name);
//Result: "Solomon Franklin"
Reflect.deleteProperty()
The Reflect.deleteProperty
method is a way to remove a property from an object. Imagine you have a toy box with toys in it, and you want to take one of the toys out. The Reflect.deleteProperty()
method is like taking a toy out of the toy box.
Here is an example:
// file: index.js
let toyBox = {
car: 'red car',
plane: 'blue plane',
boat: 'yellow boat'
};
let success = Reflect.deleteProperty(toyBox, 'car');
if(success) {
console.log('The car toy was removed successfully');
}else {
console.log('failed to remove the car toy.');
}
// in terminal
$ node index.js
The car toy was removed successfully
In this example, we have an object called toyBox
with three properties: car
, plane
, and boat
. We use the Reflect.deleteProperty()
method to remove the car
property from the toyBox
object.
This method takes in two arguments:
target
: The object from which you want to delete a property.key
: the name of the property you want to delete.
Conclusion
In this article, we learned that the Reflect API in JavaScript is a powerful tool for working with objects, providing a set of methods to perform a wide range of operations, such as
Reflect.get()
method to access the values of properties on an object.Reflect.set()
method to set the value of the property on an object.Reflect.has()
method for checking if a specified property exists or not.Reflect.delete()
method to delete a specified property from an object.Reflect.define()
method to define properties on an objectReflect.getPrototype()
method to retrieve the prototype of an object.
These capabilities allow for more flexibility and control when manipulating objects in your code, resulting in more efficient code.