Mastering the Ternary Operator, a shortcut to simplified decision-making
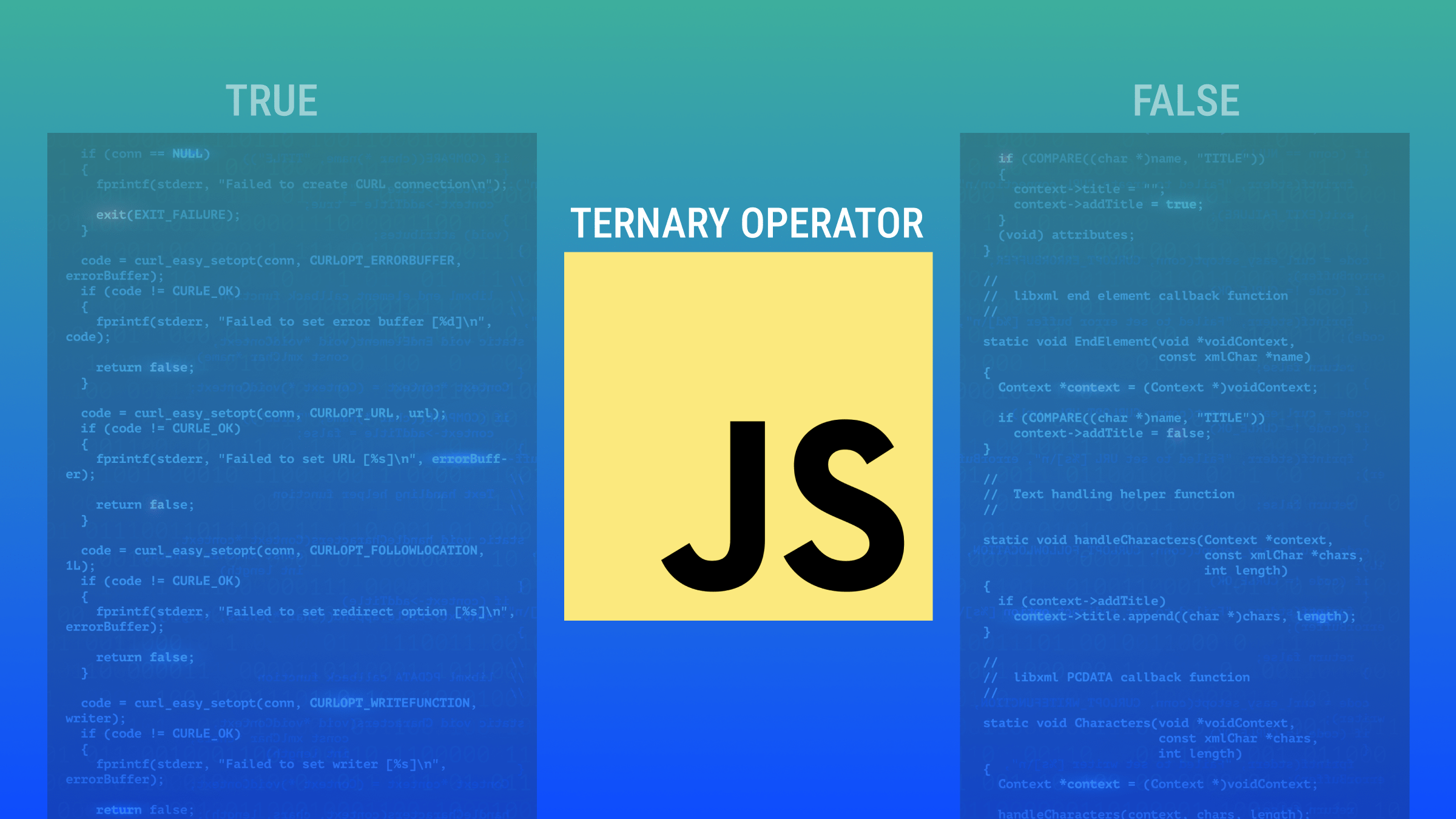
Using the ternary operator can help you write shorter, more succinct code. This article will explain it in detail and also include its possible disadvantages when used incorrectly.
Discover how at OpenReplay.com.
In the realm of JavaScript programming, the ability to make efficient decisions is paramount. Every line of code carries the weight of logic, and the way we navigate through these decisions can significantly impact the performance and readability of our applications. Enter the ternary operator, a concise and powerful tool that offers a streamlined approach to decision-making in JavaScript.
The ternary operator, denoted as condition? expression1: expression2
, provides a compact syntax for expressing conditional logic. Its succinct structure allows developers to make quick decisions within a single line of code, enhancing code readability and reducing verbosity.
In this article, we delve into the intricacies of the ternary operator, exploring its syntax, applications, and significance in modern JavaScript development. We’ll uncover how mastering this operator can serve as a shortcut to simplified decision-making, empowering developers to write cleaner, more efficient code.
Come along with us as we explore the ternary operator’s ins and outs. We’ll show you how it works and how it can improve your JavaScript coding.
Understanding the Ternary Operator
At its core, the ternary operator is a simple yet powerful construct in JavaScript, represented by the syntax condition ? expr1 : expr2
.
So, how does it work? Picture it as a shortcut for an if-else statement. Here’s the deal: the condition is evaluated first. If it’s true, expr1
gets executed; if it’s false, expr2
takes the stage. It’s like having a mini decision-making engine packed into a single line of code.
Let’s break it down with a simple example. Imagine you want to check if a number is even or odd and then display a message accordingly. Without the ternary operator, you’d probably use a traditional if-else statement. But with the ternary operator, you can achieve the same result more concisely.
const number = 10;
const message = number % 2 === 0 ? 'Even' : 'Odd';
console.log(message);
// Output: Even
In this example, number % 2 === 0
is our condition. If it is evaluated as true (which it does because 10 is indeed divisible by 2), Even
is assigned to message
. Otherwise, Odd
takes its place. And just like that, we’ve condensed our if-else statement into a neat little ternary operator, making our code cleaner and easier to understand.
Now that we’ve grasped how the ternary operator works, let’s see it in action in the real world. You’ll be surprised at just how handy this little operator can be!
Real-life Applications of the Ternary Operator
First off, let’s talk about its practical applications. The ternary operator truly shines in situations where you need to make quick decisions based on a simple condition. Think of scenarios like setting default values, toggling between two states, or even assigning CSS classes dynamically based on certain conditions.
One of the coolest things about the ternary operator is how it compares to its older cousin, the if-else statement. Sure, if-else statements get the job done, but they can be a bit verbose and clunky, especially when you’re dealing with straightforward conditions. That’s where the ternary operator swoops in to save the day, offering a more elegant and concise solution.
But let’s get down to the nitty-gritty and talk about real-world scenarios where the ternary operator can shine. Picture this: you’re building a to-do list app and want to display a checkmark icon next to completed tasks. Instead of writing out a lengthy if-else statement, you can simply use the ternary operator to toggle between displaying the checkmark and a space based on whether the task is completed or not. It’s clean, efficient, and gets the job done in just one line of code.
// Traditional if-else statement
let taskIcon;
if (task.completed) {
taskIcon = <FontAwesomeIcon icon={faCheckCircle} />;
} else {
taskIcon = <FontAwesomeIcon icon={faCircle} />;
}
// Using the ternary operator
const taskIcon = task.completed ? (
<FontAwesomeIcon icon={faCheckCircle} />
) : (
<FontAwesomeIcon icon={faCircle} />
);
This code above dynamically selects an icon (a checkmark or a circle) based on whether a task is completed. It checks the completed
property of the task object. If completed
is true, it assigns a checkmark icon; otherwise, it assigns a circle icon. The aim is to display a checkmark for completed tasks and a circle for incomplete ones. The ternary operator achieves this concisely compared to the traditional if-else statement.
Now, let’s explore some common pitfalls and traps that developers might encounter when wielding the power of the ternary operator. It’s easy to get caught up in its allure and make a few missteps, but fear not—we’re here to guide you through the maze of potential pitfalls.
Common Mistakes and Pitfalls
Despite its advantages, the ternary operator can lead to common pitfalls if not used carefully. As we journey deeper into its realm, we must be vigilant against the lurking shadows of common pitfalls.
Misunderstandings about Precedence and Associativity
It’s easy to overlook the order of operations in the heat of coding battles. Consider this:
const result = condition1 ? value1 : condition2 ? value2 : value3;
Without proper understanding, we might expect value2
to be the fallback when condition1
is false. However, due to the operator’s associativity, it’s actually equivalent to:
const result = condition1 ? value1 : (condition2 ? value2 : value3);
Always double-check the order of operations to avoid surprises.
Overuse Leads to Reduced Readability
Ah, the allure of compact code! Yet, like a double-edged sword, overusing the ternary operator can obscure our intentions. Consider this snippet:
const message = isNight ? 'Goodnight' : isMorning ? 'Good morning' : 'Hello';
While succinct, it sacrifices readability. Opt for clarity over brevity, especially for complex conditions:
let message;
if (isNight) {
message = 'Goodnight';
} else if (isMorning) {
message = 'Good morning';
} else {
message = 'Hello';
}
Best Practices for Optimal Use of the Ternary Operator
When using the ternary operator, it’s essential to adhere to best practices to ensure that your code remains readable, maintainable, and efficient. Here are some best practices to follow:
-
Keep it Simple: Use the ternary operator for simple, straightforward conditions. Avoid complex logic or nesting multiple ternary operators within each other, as it can quickly become difficult to understand.
-
Clarity Over Conciseness: Prioritize clarity in your code over brevity. Write ternary expressions that are easy to read and understand by using clear variable names and concise conditions.
-
Avoid Side Effects: Be cautious when using the ternary operator for assignments that involve side effects, such as function calls or variable mutations. Side effects within ternary expressions can lead to unexpected behavior and make the code harder to debug.
-
Use Parentheses for Clarity: When working with complex ternary expressions or combining them with other operators, use parentheses to clarify the order of operations and improve readability.
-
Consider Alternatives: While the ternary operator can be useful for simple conditional assignments, consider using traditional if-else statements or switch statements for more complex logic that requires multiple conditions or branches.
-
Add Comments for Clarity: If a ternary expression is not immediately clear from the context, consider adding comments to explain its purpose and logic. This can help future developers understand the code more easily.
-
Test Thoroughly: As with any code, thoroughly test ternary expressions to ensure they behave as expected in all possible scenarios, including edge cases and error conditions.
By following these best practices, you can leverage the ternary operator effectively in your code while maintaining readability and reducing the risk of errors.
Advanced Techniques when Using the Ternary Operator
As previously discussed, ternary operators offer a concise alternative to if…else statements. Delving deeper, advanced techniques extend their utility beyond mere replacements for simple if…else constructs. They empower developers to streamline even complex logic flows, replacing intricate if…else if…else chains and switch statements with ternary expressions. These techniques not only enhance code efficiency but also foster readability and maintainability. However, it’s important to use them judiciously to maintain code readability.
Nested Ternary Operators
Nested ternary operators are an advanced technique that consists of multiple conditional expressions nested within each other. They allow you to make decisions based on multiple conditions in a single line of code.
For example: Suppose we’re building a web application where users can sign up for different subscription plans, and we want to determine the message to display based on their subscription status:
let subscriptionLevel = "premium";
let message =
subscriptionLevel === "premium"
? "Welcome to our premium service!"
: subscriptionLevel === "standard"
? "Thank you for choosing our standard service."
: "Please sign up to access our services.";
console.log(message);
In the example above:
If the subscriptionLevel is “premium”, the message will be “Welcome to our premium service!” If the subscriptionLevel is “standard”, the message will be “Thank you for choosing our standard service.” If the subscriptionLevel is anything else, the message will be “Please sign up to access our services.
This scenario demonstrates how nested ternary operators can efficiently handle multiple conditions and determine the appropriate message to display based on the user’s subscription level.
As we draw the curtains on our exploration of the ternary operator, let’s take a moment to reflect on the journey we’ve undertaken together. From its humble beginnings as a simple conditional operator to its transformation into a powerful tool for decision-making in JavaScript, we’ve delved deep into its intricacies and uncovered its hidden potential.
Throughout this article, we’ve navigated through the basics, delving into its syntax, applications, and significance in modern JavaScript development. We’ve explored practical examples, witnessed its elegance in action, and unraveled common pitfalls and best practices to guide us on our coding endeavors.
But our journey didn’t stop there. We ventured into the realm of advanced techniques, mastering nested operators. We’ve armed ourselves with the knowledge and skills needed to wield the ternary operator with precision and confidence, unlocking its full potential as a versatile tool in our coding arsenal.
And so, as we bid farewell to the ternary operator, let us carry forward the lessons learned, the techniques mastered, and the passion ignited. May we continue to write code that is not only functional but also elegant, efficient, and a joy to behold.
Farewell, dear reader, and may your code always shine bright with the brilliance of the ternary operator. Until we meet again on our next coding odyssey, happy coding!
Complete picture for complete understanding
Capture every clue your frontend is leaving so you can instantly get to the root cause of any issue with OpenReplay — the open-source session replay tool for developers. Self-host it in minutes, and have complete control over your customer data.
Check our GitHub repo and join the thousands of developers in our community.